Webhooks
Configure webhooks in Engine to notify your backend server of transaction or backend wallet events.
Supported events
Transactions
Handle when a blockchain transaction is sent and mined onchain.
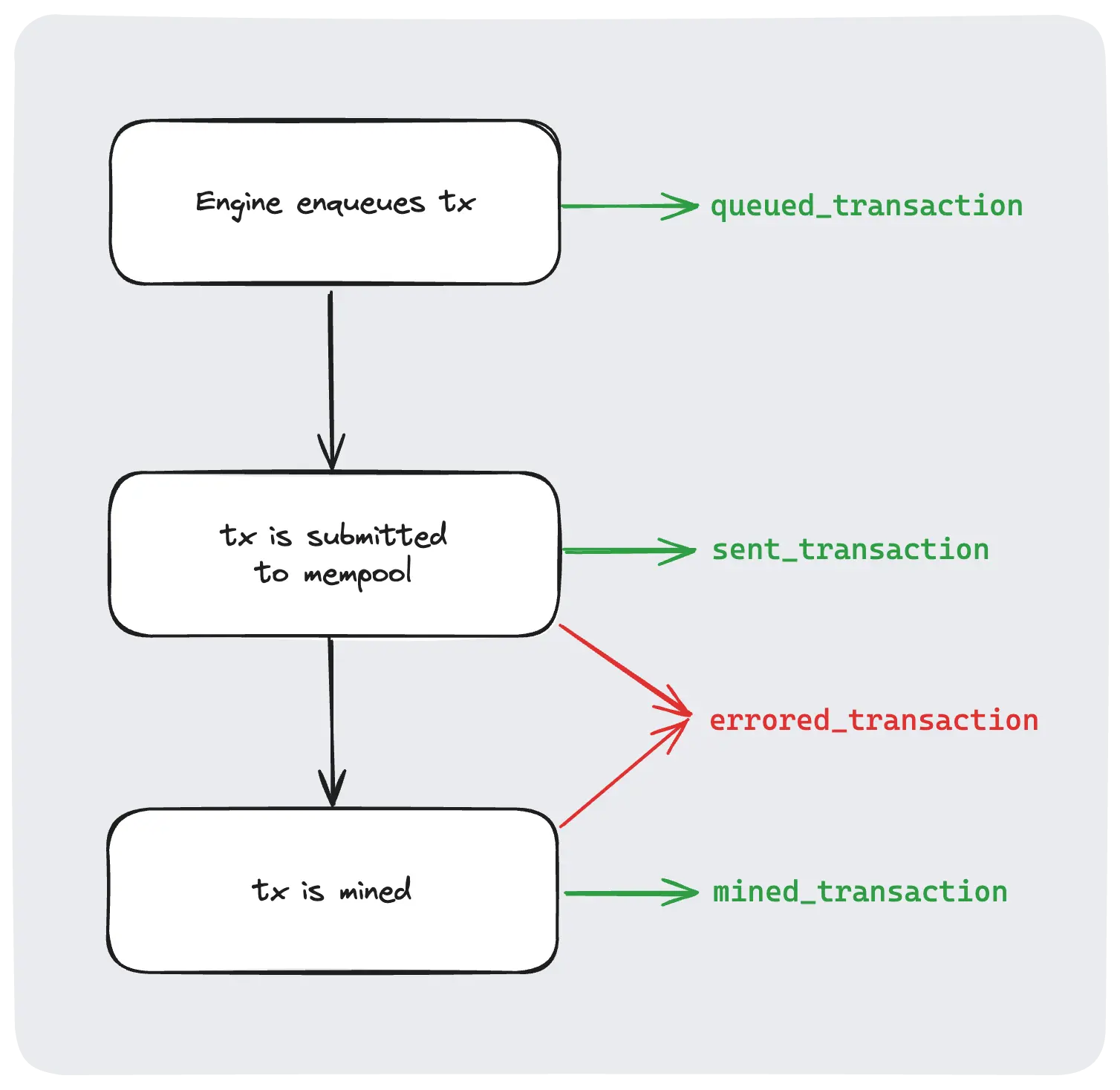
The transaction payload contains a status
field which is one of: sent
, mined
, errored
Depending on the transaction, your backend will receive one of these webhook sequences:
Note: Webhooks may come out of order. Treat later statuses as higher priority than earlier ones. For example if your backend receives a sent
webhook after a mined
webhook, treat this transaction as mined
.
Wallets
Setup
Create a webhook
- Visit the Engine dashboard.
- Select the Configuration tab.
- Select Create Webhook.
Webhook URLs must start with https://
.
Payload format
Method: POST
Headers:
Content-Type: application/json
X-Engine-Signature: <payload signature>
X-Engine-Timestamp: <Unix timestamp in seconds>
Webhook verification (recommended)
Since any outside origin can call your webhook endpoint, it is recommended to verify the webhook signature to ensure a request comes from your Engine instance.
Check the signature
The payload body is signed with the webhook secret and provided in the X-Engine-Signature
request header.
Get the webhook secret for your webhook endpoint from the dashboard.
This code example checks if the signature is valid:
Check the timestamp
The event timestamp is provided in the X-Engine-Timestamp
request header.
This code example checks if the event exceeds a given expiration duration:
Example webhook endpoint
This NodeJS code example listens for event notifications on the /webhook
endpoint: